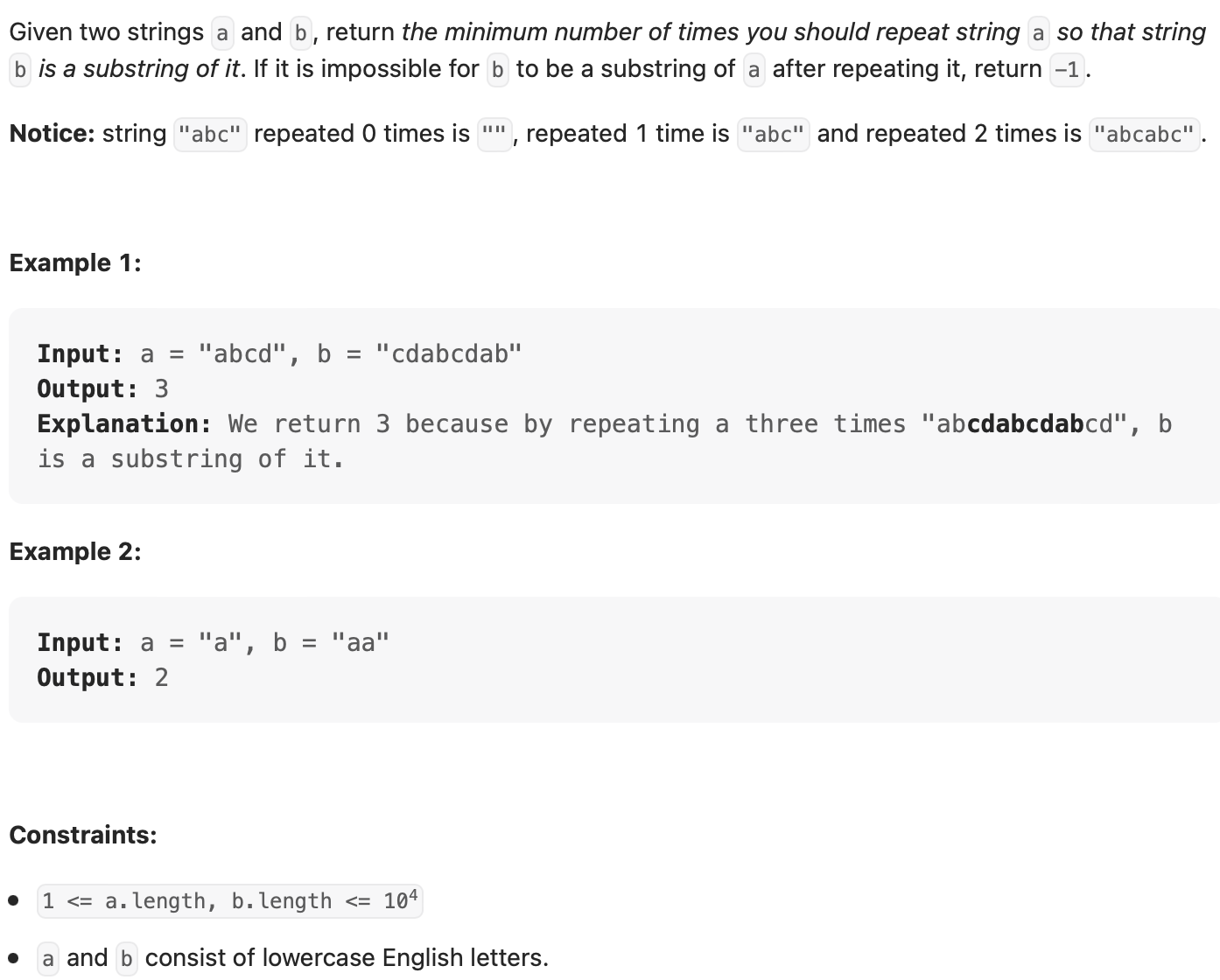
FIRST -Time Limit Exceeded
1 | public static int repeatedStringMatch(String a, String b) { |
SECOND -AC 10.57%
1 | public static int repeatedStringMatch(String a, String b) { |
THREE -AC 98.53%
1 | public static int repeatedStringMatch(String a, String b) { |
1 | public static int repeatedStringMatch(String a, String b) { |
1 | public static int repeatedStringMatch(String a, String b) { |
1 | public static int repeatedStringMatch(String a, String b) { |